The CMXads dual automatic sql and text database system all in one php script
Hi!. I thought I would provide a dual use php script example so those that use sql dont feel left out.
This script will create an sql database for use if variables are filled in for db connect.
If not it reverts to a text database system.
All the functions are included for text database read, write and delete.
All the functions are included for an sql database create, read, write and delete.
This example only writes and reads 3 data inputs but you can easily add to it.
You can enable either one you want to use.
The text database shows an example how to make a seperate file based on get value or page file name.
Its a great fast and easy way to get your project or application off to a fast start.
I hope you find it useful my friends and countrymen.
This has not been tested for bugs(I am confident there are none). But I will be setting it up as a working example real soon.
Hope you like it and use it and find it useful!
P.S. This is not what I use in my scripts. I use something even better!!
1: <?php
2: // leave these fields empty if you don't want to use a sql database:
3: //if filled in trigger sql database otherwise directory db is used
4: $db_server = ""; // server name
5: $db_user = ""; // user name
6: $db_pass = ""; // user password
7: $db_name = ""; // database name
8:
9: // sql database table and field names
10: $tbl_name = "postdata"; // table name
11: $fld_id = "ID"; // field name: ID
12: $fld_timestamp = "Timestamp"; // field name: timestamp
13: $fld_name = "Name"; // field name: name
14: $fld_email = "EMail"; // field name: e-mail
15: $fld_text = "Data"; // field name: text
16:
17: //data return order: ASC (new at bottom) or DESC (new on top)
18: $dataOrder = "DESC";
19:
20: // maximum entries to trim to and store newest
21: $dataEntries = 20;
22:
23:
24: $self = isset($_SERVER['PHP_SELF']) ? $_SERVER['PHP_SELF'] : '';
25: if(isset($_SERVER['REQUEST_URI'])){$uri = $_SERVER['REQUEST_URI'];}
26: else{
27: $uri = $self;}
28: //aquire data file name as you wish
29: //either set one file name or use uri or get to make new files
30: //example get. clean alpha num hyphen only
31: //make directory if dont exist
32: $thedir='cdata';
33: //explode file lines
34: $lineEnd = PHP_EOL;
35: $delimiter = '####';
36:
37:
38: if (!is_dir($thedir)) {
39: mkdir($thedir, 0755);
40: }
41:
42: //set your page name type to make seperate data file for each page
43: $dataFile='';
44: //if using get in uri set get value
45: //if static page name will us basename remove .php .htm .html
46:
47:
48: if(isset($_GET['id'])){
49: $getfile=preg_replace("/[^a-zA-Z0-9-]/", "", $_GET['id']);
50: $dataFile = "$thedir/$getfile.db";
51: }elseif(!isset($_GET['id']) and empty($_GET['id'])){
52: $direct=basename($uri);
53: $cat1 = str_replace(array('.php','.htm','.html'), '', $direct);
54: $dataFile = "$thedir/$cat1.db";
55: }
56: if (!file_exists($dataFile)) {
57: touch($dataFile);
58: chmod($dataFile, 0755);
59: }
60:
61:
62: // read data for text database
63: function read_data() {
64: $data = array();
65: clearstatcache();
66: global $dataFile;
67: global $lineEnd;
68: global $delimiter;
69: if(file_exists($dataFile)) {
70: if(filesize($dataFile) > 0) {
71: if($fp = fopen($dataFile, 'r')) {
72: if(flock($fp, LOCK_SH)){
73: $data = fread($fp, $size);
74: flock($fp, LOCK_UN);
75: }
76: $data = explode($lineEnd, $data);
77: for($i = 0; $i < count($data); $i++) $data[$i] = explode($delimiter, $data[$i]);
78: fclose($fp);
79: }
80: }
81: }
82: return $data;
83: }
84:
85:
86: // write data text database
87: function write_data($data) {
88: global $dataFile;
89: global $lineEnd;
90: global $delimiter;
91: if($fp = fopen($dataFile, 'w')) {
92: if(flock($fp, LOCK_EX)){
93: for($i = 0; $i < count($data); $i++) $data[$i] = implode($delimiter, $data[$i]);
94: fwrite($fp, implode($lineEnd, $data));
95: flock($fp,LOCK_UN);
96: }
97: fclose($fp);
98: }
99: }
100:
101: //builds data array to send to write_data function
102: function new_entry($name, $email, $text) {
103: global $db_name, $tbl_name, $fld_id, $fld_timestamp, $fld_name, $fld_email,
104: $fld_text, $dataEntries;
105:
106: $error = '';
107: $tstamp = date('YmdHis');
108:
109: if($db_name) {
110: if(!get_magic_quotes_gpc()) {
111: $name = addslashes($name);
112: $email = addslashes($email);
113: $text = addslashes($text);
114: }
115: $sql = "INSERT INTO $tbl_name ($fld_timestamp, $fld_name, $fld_email, $fld_text) ";
116: $sql .= "VALUES ('$tstamp', '$name', '$email', '$text')";
117:
118: if(!mysql_query($sql)) $error = mysql_error();
119: else {
120: $sql = "SELECT $fld_id FROM $tbl_name ORDER BY $fld_timestamp DESC LIMIT $dataEntries, 1";
121: $result = mysql_query($sql);
122: if(mysql_num_rows($result)) {
123: if($id = mysql_result($result, $fld_id)) {
124: $sql = "DELETE FROM $tbl_name WHERE $fld_id<=$id";
125: if(!mysql_query($sql)) $error = mysql_error();
126: }
127: }
128: }
129: }
130: else {
131: $data = read_data();
132: $len = count($data);
133: $id = $len ? $data[$len-1][0] + 1 : 1;
134: if($len >= $dataEntries) array_shift($data);
135: $data[] = array($id, $tstamp, $name, $email, $text);
136: write_data($data);
137: }
138: return $error;
139: }
140:
141:
142: // deletes from either text database or sql by id number
143: function delete_entry($id) {
144: global $db_name, $tbl_name, $fld_id;
145:
146: $error = '';
147:
148: if($db_name) {
149: if(!mysql_query("DELETE FROM $tbl_name WHERE $fld_id='$id'")) $error = mysql_error();
150: }
151: else {
152: $data = read_data();
153:
154: if(count($data)) foreach($data as $key => $val) {
155: if($val[0] == $id) {
156: array_splice($data, $key, 1);
157: write_data($data);
158: break;
159: }
160: }
161: }
162: return $error;
163: }
164:
165:
166:
167: //read data from sql if db variables set or data from read_data function
168: //sorts return array
169: function read_entries() {
170: global $msg, $db_name, $tbl_name, $fld_timestamp, $dataOrder, $dataEntries;
171:
172: if($db_name) {
173: $sql = "SELECT * FROM $tbl_name ORDER BY $fld_timestamp $dataOrder LIMIT $dataEntries";
174: $result = mysql_query($sql);
175: while($row = mysql_fetch_row($result)) $data[] = $row;
176: }
177: else {
178: $data = read_data();
179: if(strtoupper($dataOrder) != 'ASC') rsort($data);
180: }
181: return $data;
182: }
183:
184:
185: //example process return data from read_entries as desired
186: for($i = 0; $i < count($data); $i++) {
187: $id = $data[$i][0];
188: $tstamp = $data[$i][1];
189: $name = $data[$i][2];
190: $email = strstr($data[$i][3], '@') ? $data[$i][3] : '';
191: $text = nl2br($data[$i][4]);
192: }
193: ///////////////////////////////// sql
194: function db_open($db_server, $db_user, $db_pass, $db_name) {
195: $status = true;
196:
197: if(!@mysql_connect($db_server, $db_user, $db_pass)) {
198: sql_error();
199: $status = false;
200: }
201: else if(!@mysql_select_db($db_name)) {
202: sql_error();
203: $status = false;
204: }
205: return $status;
206: }
207:
208: function sql_error() {
209: echo '<span style="color:red;">' . mysql_errno() . ': ' . mysql_error() . '</span><br>';
210: }
211:
212:
213: if(!$db_name || db_open($db_server, $db_user, $db_pass, $db_name)) {
214: $table_exists = true;
215: }
216: //if sql is set and cant open table
217: if($db_name) {
218: if(!mysql_query("SELECT 1 FROM $tbl_name LIMIT 1")) $table_exists = false;
219: $create ="yes";
220: }
221:
222:
223: if($db_name && !$table_exists) {
224:
225: if($create == 'yes') {
226: $sql = "CREATE TABLE $tbl_name ( " .
227: "$fld_id INT(10) NOT NULL auto_increment, " .
228: "$fld_timestamp VARCHAR(14) NOT NULL, " .
229: "$fld_name VARCHAR(20), " .
230: "$fld_email VARCHAR(75), " .
231: "$fld_text TEXT NOT NULL, " .
232: "PRIMARY KEY ($fld_id))";
233: if(!mysql_query($sql)) $error = mysql_error();
234: }
235: }
236:
237: if($db_name) mysql_close();
238:
239: ?>
free download
Any modifications you would like are a 10cents each task.
Please help me I am handicapped and support myself
Donate With PayPal
Donate Bitcoin
Please donate and help the handicapped.
1KtuX6N6xM2YxijE7JfPeHZmtGKGQwgfpc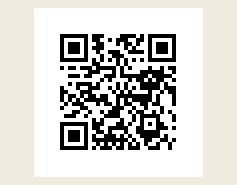
Thank you you are the best. Works like a charm. I sent you 22 cents.
name:Krysten Date:08.7.22 @ 06:21am IP:83.1049.1.2711
Thanks for sharing.
name:Krysten Date:08.7.22 @ 06:21am IP:31814.91.107.2
Thanks for sharing.
name:Doloris Date:01.21.19 @ 11:42am IP:5.188.211.26
Thanks for sharing.
name:Precious Date:01.20.19 @ 01:35am IP:5.188.211.15
Worked out great for me I sent you 29 cents.
name:Krysten Date:10.16.18 @ 03:54am IP:5.188.210.11
Worked out great for me I sent you 29 cents.
name:Doloris Date:10.11.18 @ 18:46pm IP:5.188.210.11
Thanks I admire you I sent you 67 cents.
name:Judas Date:02.23.18 @ 04:10am IP:146.185.223.252
Thanks you are awesome I sent you 6 cents.